Mastering JavaScript: Expert-Level Assignments and Solutions
Welcome, aspiring JavaScript developers and enthusiasts! In today's post, we delve into the intricate world of JavaScript assignments, exploring challenging problems that will sharpen your coding skills and deepen your understanding of this versatile language. Whether you're a student seeking guidance or a seasoned programmer looking to refine your craft, you're in the right place.
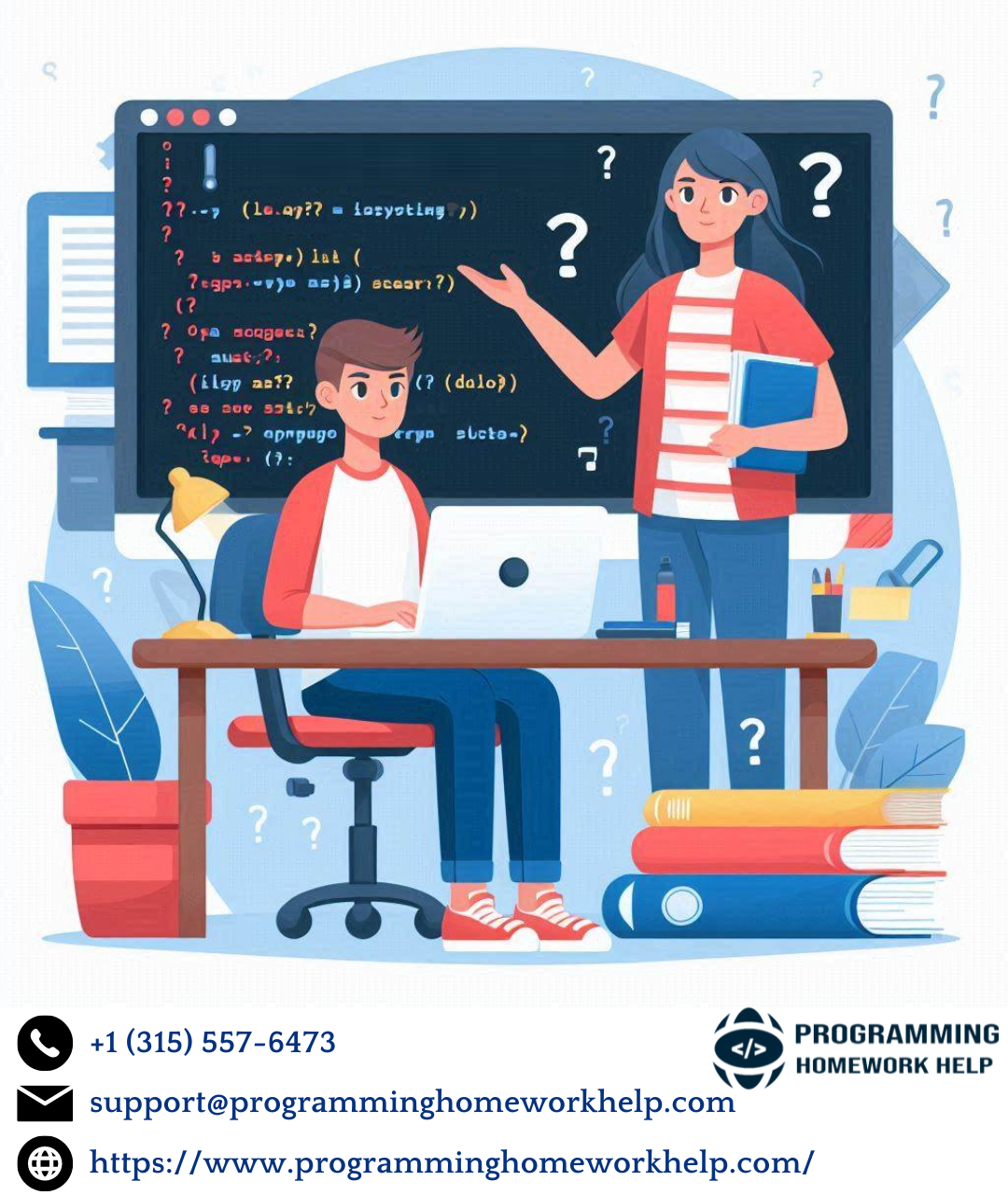
At ProgrammingHomeworkHelp.com, we specialize in providing comprehensive JavaScript assignment help tailored to your needs. Our team of experts excels in dissecting complex problems, offering insightful solutions, and guiding you through every step of the learning process. So, let's dive in and tackle some master-level JavaScript questions together!
Question 1: Object-Oriented Programming (OOP) Challenge
You're tasked with implementing a simple banking system using JavaScript's object-oriented programming principles. Create a class named BankAccount
with the following properties and methods:
Properties:
owner
: The name of the account owner.balance
: The current balance of the account.
Methods:
deposit(amount)
: Adds the specified amount to the account balance.withdraw(amount)
: Subtracts the specified amount from the account balance. Ensure that the withdrawal amount does not exceed the available balance.getBalance()
: Returns the current balance of the account.
Solution:
class BankAccount {
constructor(owner, balance) {
this.owner = owner;
this.balance = balance;
}
deposit(amount) {
this.balance += amount;
console.log(`${amount} deposited. Current balance: ${this.balance}`);
}
withdraw(amount) {
if (amount > this.balance) {
console.log("Insufficient funds");
} else {
this.balance -= amount;
console.log(`${amount} withdrawn. Current balance: ${this.balance}`);
}
}
getBalance() {
console.log(`Current balance: ${this.balance}`);
return this.balance;
}
}
// Example Usage:
const myAccount = new BankAccount("John Doe", 1000);
myAccount.deposit(500);
myAccount.withdraw(200);
myAccount.getBalance();
Question 2: Asynchronous Programming Puzzle
You're building a web application that fetches data from an external API and displays it on the page. However, the API endpoints have rate limits, and you need to implement a solution to handle asynchronous requests gracefully. Write a function named fetchData
that fetches data from the API with the following requirements:
The function should accept a URL parameter indicating the API endpoint.
Implement a retry mechanism that retries the request with exponential backoff if the API returns a rate limit exceeded error (HTTP status code 429).
Once the data is successfully fetched, log it to the console.
Solution:
async function fetchData(url, retries = 3, delay = 1000) {
try {
const response = await fetch(url);
const data = await response.json();
console.log("Data fetched successfully:", data);
} catch (error) {
if (retries === 0 || error.status !== 429) {
console.error("Failed to fetch data:", error.message);
return;
}
console.log("Rate limit exceeded. Retrying...");
await new Promise((resolve) => setTimeout(resolve, delay));
await fetchData(url, retries - 1, delay * 2);
}
}
// Example Usage:
const apiUrl = "https://api.example.com/data";
fetchData(apiUrl);
Conclusion
Mastering JavaScript involves not only understanding the syntax but also mastering its core concepts and applying them to solve real-world problems. Through challenging assignments like the ones presented here, you can hone your skills and become a proficient JavaScript developer.
At ProgrammingHomeworkHelp.com, we're dedicated to providing top-notch JavaScript assignment help to students and professionals alike. Whether you're struggling with object-oriented programming or asynchronous requests, our experts are here to guide you every step of the way.
Keep coding, keep learning, and never hesitate to seek assistance when needed. Together, we'll unlock the full potential of JavaScript and pave the way for a brighter future in programming. Happy coding!